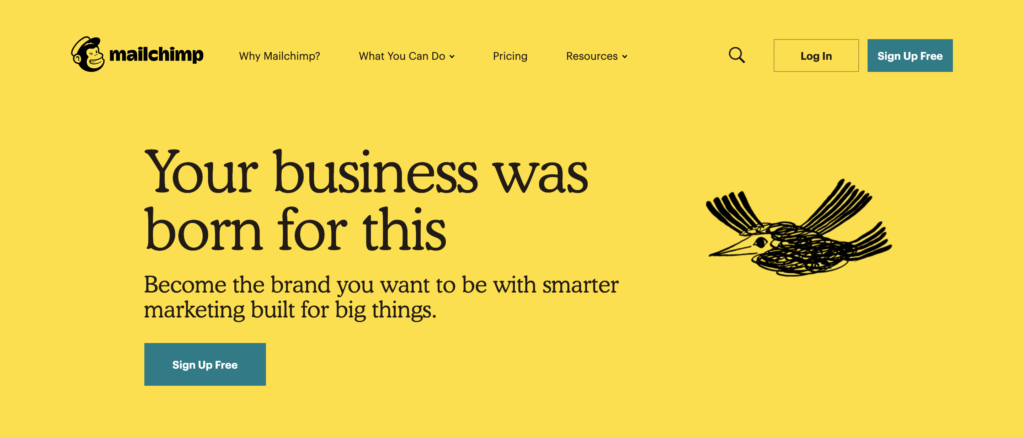
Step 1: Create a Basic Form
This basic form will be used to submit details to the MailChimp API. At the end of this tutorial, the source code is available as a downloadable ZIP. However, I would advise going through this tutorial step-by-step first.
<form action="" method="post">
<label class="field">
Name
<input name="name" type="text" value="" />
</label>
<label class="field">
Email
<input name="email" type="email" value="" />
</label>
<input type="submit" />
</form>
Step 2: Connecting to the MailChimp API using a PHP Wrapper
The MailChimp API PHP wrapper that I use can be found at https://github.com/drewm/mailchimp-api
Firstly, we need to make a connection to the relevant file inside the MailChimp PHP wrapper.
In this case, we are going to submit a user’s details to MailChimp that will subscribe them to our list. To do this a connection to the csrest_subscribers.php file is necessary. Depending on your directory structure the following line might differ.
<?php require_once( __DIR__. '/../libraries/mailchimp/src/MailChimp.php');?>
Next, we will need to set up a function that will be used to submit the form details to MailChimp.
<?php function storeAddress($first,$last,$email) {
$key = 'List ID';
$list_id = 'API Key';
$merge_vars = array(
'FNAME' => $first,
'LNAME' => $last
);
$mc = new MailChimp($key);
// add the email to your list
$result = $mc->post('/lists/'.$list_id.'/members', array(
'email_address' => $email,
'merge_fields' => $merge_vars,
'status' => 'subscribed'
));
return json_encode($result);
};?>
In the above code, you will notice that the List ID and API Key will need to be replaced and these can be found when you log into your MailChimp Account.
Step 3: Connecting the Form to the API Connection
This stage is all down to preference and the way in which you handle form submissions. This can be written using Javascript or PHP. In this simple case, we are going to use PHP to submit the details on page reload.
<?php function do_newsletter_response () {
// Exit early if page render is not a POST request
if ($_SERVER['REQUEST_METHOD'] != 'POST') return;
storeAddress($_POST['first_name'],$_POST['last_name'],$_POST['email']);
}
do_newsletter_response();?>
Conclusion
This tutorial has displayed the basics on how to connect to the MailChimp API. This tutorial is only the beginning of what is achievable with the MailChimp API. An apparent improvement on what we have written here is form validation. However, this will be available as another tutorial in the future.
The source code for this tutorial will be available soon.