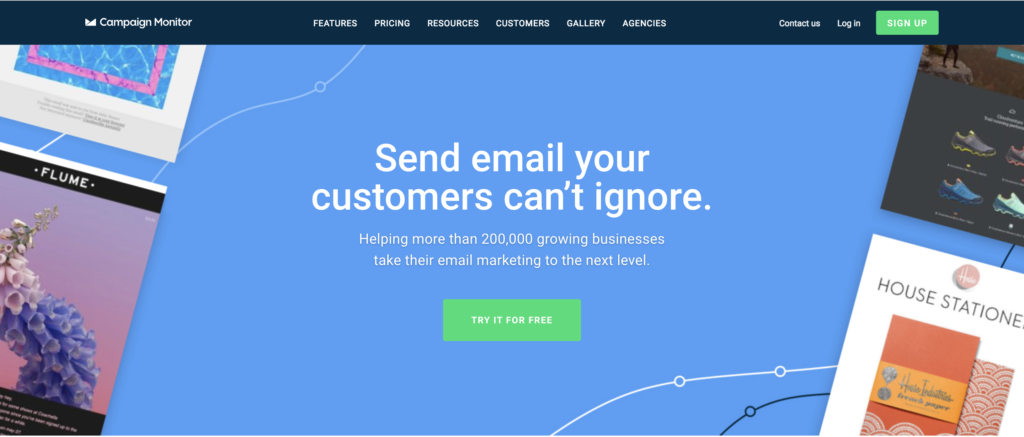
Step 1: Create a Basic Form
This basic form will be used to submit details to the Campaign Monitor API. At the end of this tutorial, the source code will be supplied as a downloadable ZIP. However, I would advise going through this tutorial step-by-step first.
<form action="" method="post">
<label class="field">
Name
<input name="name" type="text" value="" />
</label>
<label class="field">
Email
<input name="email" type="email" value="" />
</label>
<input type="submit" />
</form>
Step 2: Connecting to the Campaign Monitor API using a PHP Wrapper
The Campaign Monitor API PHP wrapper can be found at http://campaignmonitor.github.io/createsend-php/.
Firstly, we need to make a connection to the relevant file inside the campaign monitor PHP wrapper.
In this case, we are going to submit a user’s details to campaign monitor that will subscribe them to our list. To do this a connection to the csrest_subscribers.php file is necessary. Depending on your directory structure the following line might differ.
<?php require_once( __DIR__. '/../libraries/campaignmonitor/csrest_subscribers.php');?>
Next, we will need to set up a function that will be used to submit the form details to campaign monitor.
<?php function storeAddress($name,$email) {
$wrap = new CS_REST_Subscribers('List ID', 'API Key');
$result = $wrap->add(array(
'EmailAddress' => $email,
'Name' => $name,
'Resubscribe' => true
));
};?>
In the above code, you will notice that the List ID and API Key will need to be replaced and these can be found when you log into your Campaign Monitor Account.
Step 3: Connecting the Form to the API Connection
This stage is all down to preference and the way in which you handle form submissions. This can be written using javascript or PHP. In this simple case, we are going to use PHP to submit the details on page reload.
<?php function do_newsletter_response () {
// Exit early if page render is not a POST request
if ($_SERVER['REQUEST_METHOD'] != 'POST') return;
storeAddress($_POST['name'],$_POST['email']);
}
do_newsletter_response();?>
Conclusion
This tutorial has displayed the basics on how to connect to the Campaign Monitor API. This is only the beginning of what is achievable with the Campaign Monitor API. An apparent improvement on what we have written here is form validation. This will be left for another tutorial in the future.
The source code for this tutorial can be downloaded here.